Front-end developers spend a lot of time fixing script errors. While researching cross-browser compatibility issues in JavaScript last month, we discovered that JavaScript errors are always present, no matter how well-written the code is. In the past, errors could be inspected with console.log and alert ().
The developer could eventually find the source of the error by adding them to every line of code. This was very time-consuming. It was like asking a sculptor for a large-sized stone statue to be carved using a pen knife.
Almost all major browsers have built-in developer tools, thanks to the latest browser updates. This article will show you how to quickly debug complex issues in JavaScript automation testing using the developer console.
In most browsers that use the Windows platform, the developer’s tool can be accessed using F12 or Ctrl+Shift+i. Or by right-clicking on an element to navigate to Inspect Element. You can access the developer tools in your Mac browser by pressing Cmd+Opt+i. You can find the script debugger under the Sources tab. Developers can inspect the code without the need for alert popups by using the script debugger’s breakpoints.
Let’s consider a scenario in which your JavaScript code throws an exception. Chrome users should enable the “Pause On Exceptions” icon. Allowing it will stop code execution when an exception is caught. Code execution will not be stopped if the library handles the exception. The feature is only available in Firefox or Chrome, not in IE.
Contents
When Inspecting The Code
Now that execution has halted, we can inspect the code and determine what is going wrong. This is the most significant benefit of using the debugger. The script can access everything the developer needs.
The code view shows that the exception line is marked with a red icon. You can either hover on the icon to see the error message or view the console error message. You can inspect the variables by hovering your mouse over them. You can also find additional information under the scope tab. Variables are arranged by scope in the scope tab. All local variables, for example, are placed under the “local” section.
Rectifying The Problem
If there are minor errors, the error message will give us an idea of why. If forEach is used, and the URL has an invalid value, we will receive an error message saying, “forEach cannot be a function.” You can fix the bug by using an if clause and checking the variable type. However, a front-end developer with experience will be able to identify and fix the problem’s root cause.
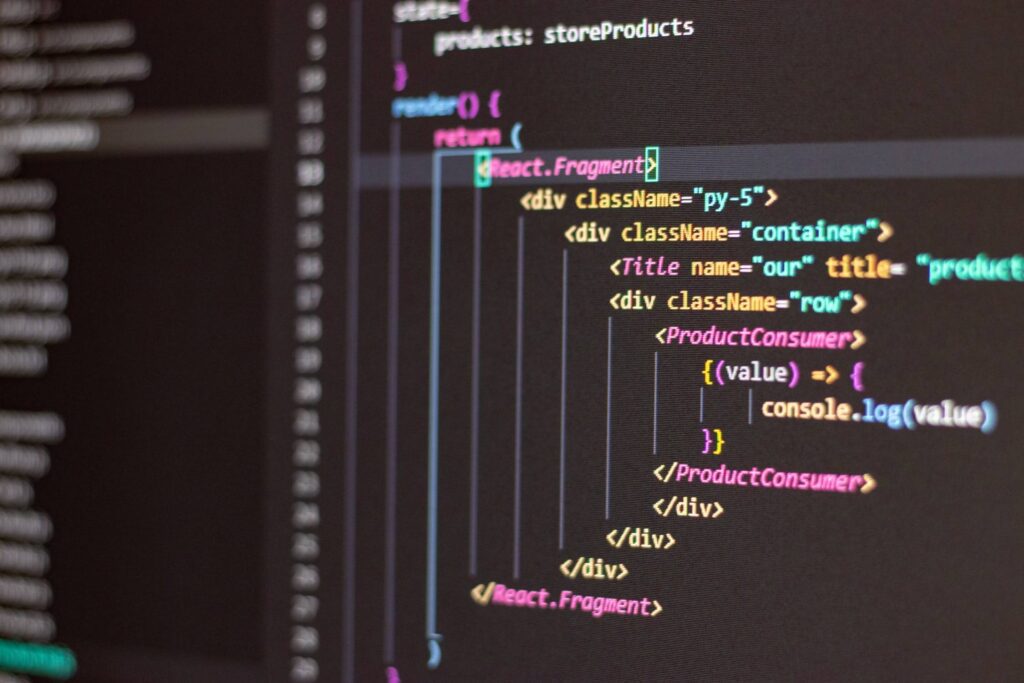
Analyzing The Code From Root
You can search the Call Stack tab to find the root cause. Call Stack displays the parent elements of each function being inspected. It displays a list of functions. All previous functions can be accessed by clicking on the list. You should inspect the parent functions until you find the root cause. The search for the source of the error is complete once we have located it.
Debugging Complex Issues Using Breakpoints
Breakpoints are a feature of the script debugger that allows developers to solve more complex problems. You can set breakpoints by adding a debugger statement inline or clicking on the line number at the script debugger.
When we add the debugger, click the “run.” Code execution begins, and the debugger stops execution at the line containing debugger statements. Breakpoints should always be added to every function until the line containing the error is found.
Script errors that are detected while debugging will be displayed on the console. Only one job remains for the developer. You must identify the error line, fix it, and then debug the code again.
Debugging Using Console
Developers can use certain commands within the console to fix performance problems in addition to the inbuilt debugging tools.
- console.time() is used to set a countdown. console.timeEnd() can print the value. This will show you how long it took to execute a function.
- To find a more difficult performance issue, a console can be used. Displays the heap size.
- console.count() is useful for determining how often the script has read your code.
- If you want to log a value when the condition is false, console.assert(condition, msg) can be used. This may cause an Assertion error when using Node JS.
- console.table() allows you to display the objects that have been logged in a neatly arranged table format.
- Clear() is used to clear logs from your console.
A browser’s built-in debugging tool is powerful enough to reduce the time required to debug code. Your browser should be updated to make debugging easier and more fun, regardless of whether you use the console or script debugger.
Debugging Complex Issues In JavaScript Automation Testing Using LambdaTest
JavaScript isn’t cross-browser compatible. This is a hard truth. All browsers do not support JavaScript. Older browsers do not support some of the most commonly used JS tags. It can be difficult to find cross-browser compatibility issues and fix them.
LambdaTest offers a platform that supports 3000+ operating system and browser combinations to test your applications using automation tools like Selenium, Playwright, or Appium. Each one is equipped with powerful debugging tools. This platform is ideal for debugging cross-browser compatibility issues, especially when you cannot access the browser version or operating system. You can also use our mobile browser debugging tools to solve mobile web problems.